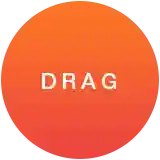
In recent years, the demand for mobile applications has skyrocketed, driven by the increasing reliance on smartphones for various tasks. As a result, developers are constantly exploring new frameworks and tools to streamline the app development process. One such powerful framework that has gained popularity is Next.js. Originally designed for building React applications, Next.js has become a versatile framework for building Next.js mobile apps.
With the rise of the Next.js mobile app ecosystem, developers can leverage its capabilities to create robust and scalable mobile applications.
What is Next.js?
Next.js for mobile app is a React framework that allows developers to build web applications quickly. It simplifies the React development process by providing a set of conventions and tools that enhance productivity. When starting a project with Next.js, developers can initiate the setup using the "next js install" command, streamlining the installation process.
Additionally, Next.js databases allow developers to manage data in their applications efficiently. With features such as server-side rendering and API routes, Next.js facilitates smooth database interactions, making it a robust choice for projects requiring dynamic content. Whether you're working with MongoDB, MySQL, or other databases, Next.js provides a flexible environment to handle data and build powerful web applications.
When you use the next create app command, it sets up a new project with all the necessary configurations, allowing you to focus on developing your application with a well-structured foundation.
Key features of Next.js include:
- Server-Side Rendering (SSR): Next.js enables SSR by default, allowing the server to render the initial HTML content. This leads to accelerated page loading times and enhanced search engine optimization (SEO).
- Static Site Generation (SSG): With Next.js, you can pre-render pages at build time, generating static HTML files. This approach is beneficial for performance and scalability.
- Automatic Code Splitting: Next.js automatically splits your code into smaller chunks, ensuring that only the necessary code is loaded for each page. This enhances performance by reducing the initial page load time.
- File-Based Routing: Next.js simplifies routing by following a file-based convention. Each JavaScript file in the 'pages' directory corresponds to a specific route within the application.
- CSS-in-JS Support: Next.js supports various CSS-in-JS solutions, making it easy to style your components and manage stylesheets effectively. Whether building a Next.js website or a Next.js full-stack application, the framework provides a robust and efficient development environment, empowering developers to create dynamic and responsive web applications.
Setting Up Your Next.js Project
You must set up your project to start building a Next.js mobile app with Next.js. Follow these steps to create a basic Next.js app:
Start by using the nextjs create app command to initialize your project, which will set up the necessary structure and dependencies for your development.
- Install Node.js and npm:
Ensure that Node.js and npm are installed on your machine. You can obtain and install them directly from the Node.js website.
- Create a Next.js App:
Open your terminal and run the following commands to create a new Next.js app:
This will create a new Next.js app in a directory named 'my-mobile-app.'
- Run the Development Server:
Start the development server with the following command:
Your Next.js app should now be running locally at http://localhost:3000. Open this URL in your browser to see the default Next.js landing page.
Adding Mobile Responsiveness
Mobile responsiveness is crucial for a successful mobile app. Next.js, built on React, makes it relatively straightforward to ensure your app looks great on various devices. Here are some best practices for adding mobile responsiveness to your Next.js app:
- Viewport Meta Tag:
Insert the viewport meta tag to your ‘head’ element in the ‘pages/_app.js’ file. This tag is essential for controlling the width and scaling of the viewport on different devices.
- Responsive Styles:
Use media queries in your CSS or styling solution to define styles for different screen sizes. For example, you can create a ‘styles/mobile.css’ file and import it where needed:
- Testing on Multiple Devices:
Regularly conduct testing on a range of devices and browsers to guarantee a uniform and optimal user experience, especially for applications like a B2B travel app. Browser developer tools often include device emulators that simulate different screen sizes.
Implementing Navigation
Effective navigation is a pivotal element in any mobile application. Next.js streamlines the navigation process through its integrated routing system. Here's how you can implement navigation in your mobile app:
- Link Component:
Use the ‘Link’ component from Next.js to create navigation links. Import it and use it as follows:
- Programmatic Navigation:
You can also perform programmatic navigation using the ‘useRouter’ hook from ‘next/router’. For example, redirecting the user to another page after a button click:
Handling State Management
State management is crucial to building mobile apps, especially those with complex user interfaces. Next.js, being a React framework, supports various state management solutions. One popular choice is using the ‘useState’ hook for local component state and a global state management library like Redux for managing application-wide state.
- Local Component State:
Use the ‘useState’ hook to manage the local component state. For example, creating a simple counter component:
- Global State Management:
For managing the global state, consider using a library like Redux. Install the necessary packages and set up your store:
Integrate the Redux store with your app:
Access the global state in your components using the ‘useSelector’ hook:
Integrating API Calls
Many mobile apps require interaction with APIs to fetch and update data. Next.js provides a straightforward way to make API calls, whether they are server-side or client-side.
- Server-Side API Calls:
Use the ‘getServerSideProps’ function to fetch data on the server side and pass it as props to your component. For example, fetching data from an API:
- Client-Side API Calls:
Use the ‘useEffect hook’ and the ‘fetch’ API to make client-side API calls. For example, fetching data when the component mounts:
Optimizing Performance
Enhancing the performance of your mobile application is crucial for providing a smooth user experience. Next.js offers several features and best practices to enhance performance.
- Image Optimization:
Use the ‘next/image’ component for image optimization. It automatically optimizes images and provides features like lazy loading and automatic resizing.
- Code Splitting:
Leverage Next.js's automatic code splitting to ensure that only the necessary code is loaded for each page. This can significantly reduce decrease the time it takes for the initial page to load.
- Bundle Analysis:
Use tools like ‘next-bundle-analyzer’ to analyze your app's bundle size. Identify and optimize large dependencies to reduce the overall size of your application.
Update your ‘next.config.js’ file to use the bundle analyzer:
Run the bundle analyzer:
- Caching and CDN:
Implement caching strategies and consider using a Content Delivery Network (CDN) to cache static assets. This can substantially decrease server load and enhance response times.
Building and Deploying Your Mobile App
Once you've developed your mobile app with Next.js, it's time to build, deploy, and even explore ways to convert your Next.js application to a mobile app. Ensure that your application is production-ready by following these steps:
- Build the App:
Run the following command to build your Next.js app for production:
This command generates an optimized production-ready build in the out directory.
- Deploy to a Hosting Service:
Select a hosting service for your mobile app. Popular options include Vercel, Netlify, and AWS Amplify. These platforms offer seamless integration with Next.js and make the deployment process straightforward.
For example, deploying to Vercel involves connecting your GitHub repository and configuring the deployment settings. Once set up, your app will automatically deploy whenever you push changes to the repository.
- Set Up Continuous Integration/Continuous Deployment (CI/CD):
Consider implementing CI/CD pipelines to automate the deployment process. This ensures that your app is consistently deployed and updated without manual intervention.
- Monitor and Analyze:
Leverage monitoring tools and analytics to track the performance and usage of your mobile app. This information can help you identify areas for improvement and ensure a positive user experience.
Conclusion
Building a mobile app with Next.js provides a powerful and efficient development experience. You can easily create high-performance mobile applications by leveraging its features, such as server-side rendering, automatic code splitting, and built-in routing. Additionally, integrating mobile responsiveness, state management, API calls, and optimizing performance ensures a seamless user experience.
Using the nextjs create app command helps streamline the setup process, providing a solid foundation to build and optimize your mobile app effectively.
Let’s compare Next.js with other frameworks:
- Next.js vs Remix: Choose based on specific project requirements, Next.js is known for ease of use and server-side rendering, while Remix emphasizes enhanced developer experience.
- Node.js vs Next.js: Node.js is a runtime enabling server-side JavaScript, while Next.js is a framework built on Node.js, providing a structured approach for web applications with server-side rendering needs.
- Gatsby vs Next.js: Choose based on specific project requirements, Next.js is known for ease of use and server-side rendering, while Remix emphasizes enhanced developer experience.
- Next.js vs Express: Express is a minimal Node.js framework for APIs and routing, while Next.js, a higher-level framework, is designed for React applications with server-side rendering. Choose based on project needs and desired features.
- With the right tools and knowledge, you'll be well-equipped to create feature-rich and performant mobile applications that meet the demands of today's users. At Saffron Tech, we specialize in guiding you on when to use Next.js for building top-notch mobile applications using Next.js. We have the tools and knowledge to help you choose the best CMS for Next.js and create feature-rich and performant mobile applications that meet the demands of today's users.
- If you're curious about how to create mobile apps with JavaScript or want to explore how to use Next.js to mobile app development, we can provide expert advice and practical solutions to help you leverage JavaScript's capabilities for your mobile app development needs. Contact us today to learn more!